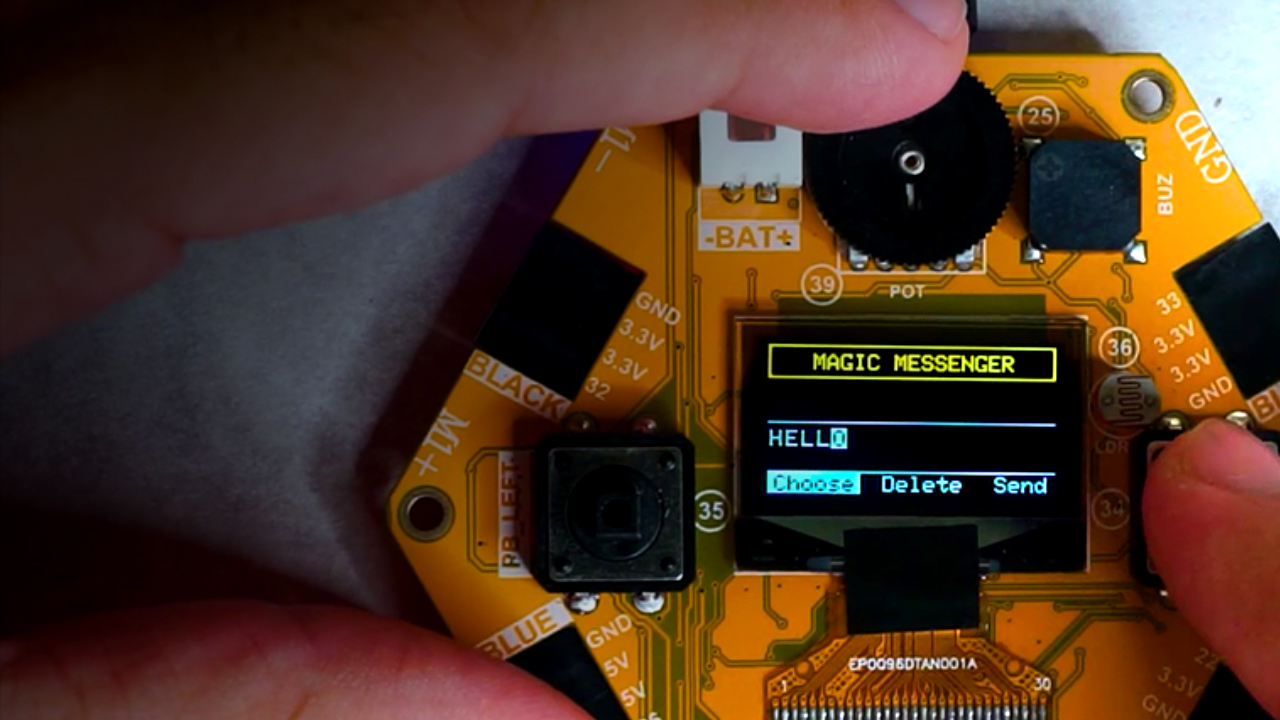
Magic Messenger
Components Required
Introduction
In the magic messenger project, we will use two Magicbit boards and communicate between them. We will make a separate user interface for this purpose. The potentiometer and buttons on the board will be used as input mechanisms. The communication will be done using ESP-NOW.
ESP NOW? What is it?
ESP now is a protocol by Espressif. It enables multiple devices to communicate with one another using Wi-Fi. The protocol is similar to the low-power 2.4Ghz wireless connectivity. The devices are needed to be paired before the communication can happen. There is no need for a handshake after the pairing is done. (safe and peer-to-peer).
What You Need
- Two Magicbit boards
Method
You have to know the MAC addresses for your two boards. It is a unique number given to the board. Upload the below code to the Magicbit boards seperately. Check the serial monitor for a MAC address. Copy down these addresses separately as you will need them later.
# Run this code to find the MAC adress #include "WiFi.h" void setup(){ Â Serial.begin(115200); Â WiFi.mode(WIFI_MODE_STA); Â Serial.println(WiFi.macAddress()); } void loop(){ }
When you have the addresses, note them down. Let me label the MAC addresses as shown below, so it is easy to explain.
Now copy the below code and paste it into your Arduino IDE.
// Code 2 // By MagicBit #include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #include <esp_now.h> #include <WiFi.h> esp_now_peer_info_t peerInfo; #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels // Declaration for an SSD1306 display connected to I2C (SDA, SCL pins) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // Use the MAC of the other board below uint8_t broadcastAddress[] = {0x78, 0x21, 0x84, 0x8D, 0xFF, 0x50}; int choose = 0; int inputLine_y = 36; int inputSpace_x = 7; int R_count = 0; int potValue; char Msg[27]={' '}; char got[18]; char sent[18]; char elements[] = " ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"; void OnDataRecv(const uint8_t * mac, const uint8_t *incomingData, int len) {  memcpy(&got, incomingData, sizeof(got));  Serial.print("Bytes received: ");  Serial.println(len);  Serial.print("Message: ");  Serial.println(got);   for (int i=0; i<18;++i) {    display.fillRect(i*7,18, 7, 9, BLACK);  }   display.setCursor(1,19);   // input  display.setTextColor(WHITE);  display.println(got);  } void setup() {  Serial.begin(115200); //===============================  WiFi.mode(WIFI_STA);  if (esp_now_init() != ESP_OK) {    Serial.println("Error initializing ESP-NOW");    return;  }   memcpy(peerInfo.peer_addr, broadcastAddress, 6);  peerInfo.channel = 0;   peerInfo.encrypt = false;    if (esp_now_add_peer(&peerInfo) != ESP_OK){    Serial.println("Failed to add peer");    return;  }  esp_now_register_recv_cb(OnDataRecv);  //===============================  if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { // Address 0x3D for 128x64    Serial.println(F("SSD1306 allocation failed"));    for(;;);  }  delay(2000);  display.clearDisplay();  display.drawRect(0, 0, 128, 15, 1);  display.setTextSize(1.5);  display.setTextColor(WHITE);  display.setCursor(20,4);  display.print("MAGIC MESSENGER");  display.drawLine(0,54,127,54,WHITE);  display.display();  display.drawLine(0,32 , 127, inputLine_y-4, WHITE);   pinMode(34,INPUT);  pinMode(35,INPUT);  pinMode(39,INPUT); } void loop() {  Serial.println(Msg);   potValue = analogRead(39);  int Lbutton = digitalRead(35);  int Rbutton = digitalRead(34);  if ((Rbutton==LOW)&&(choose==0)) {   // For Choose    delay(300);    Msg[R_count] = elements[map(potValue)];    ++R_count;  }   if ((Rbutton==LOW)&&(choose==1)) {   // Delete all    delay(300);    R_count = 0;    //strcpy(Msg,"");    char Msg_tmp[27]={' '};    strcpy(Msg,Msg_tmp);       for (int i=0; i<18;++i) {      display.fillRect(inputSpace_x * i, inputLine_y - 1, 7, 9, BLACK);    }  }  if ((Rbutton==LOW)&&(choose==2)) {    delay(300);    strcpy(sent, Msg);    esp_err_t result = esp_now_send(broadcastAddress, (uint8_t *) &sent, sizeof(sent));      if (result == ESP_OK) {      Serial.println("Sent with success");    }    else {      Serial.println("Error sending the data");    }  } //==============================  if (Lbutton == LOW){    delay(300);    choose++;       if (choose > 2) {      choose = 0;    }  }  menu(choose); //==============================  display.fillRect(inputSpace_x * R_count, inputLine_y, 5, 7, BLACK);  display.fillRect(inputSpace_x * R_count, inputLine_y - 1, 7, 9, WHITE);  display.setCursor(inputSpace_x * R_count + 1, inputLine_y);  display.setTextColor(BLACK);   display.println(elements[map(potValue)]);  for (int i=0; i<R_count;++i) {    display.fillRect(inputSpace_x * i, inputLine_y - 1, 7, 9, BLACK);    display.setCursor(inputSpace_x*i +1, inputLine_y);   // input    display.setTextColor(WHITE);    display.println(Msg[i]);  }  display.display(); } void menu(int pos) {  switch (pos) {    case 0 :{    display.fillRect(48, 55, 45, 9, BLACK); //delete    display.fillRect(98, 55, 30, 9, BLACK); //SEND       display.fillRect(0, 55, 41, 9, WHITE);    display.setTextSize(1);    display.setTextColor(BLACK);    display.setCursor(3, 56);    display.println("Choose");    display.setTextSize(1);    display.setTextColor(WHITE);    display.setCursor(51, 56);    display.println("Delete");    display.setTextSize(1);    display.setTextColor(WHITE);    display.setCursor(101, 56);    display.println("Send");     display.display();    }    break;    case 1 :{    display.fillRect(0, 55, 41, 9, BLACK); //choose    display.fillRect(98, 55, 30, 9, BLACK); //SEND       display.setTextSize(1);    display.setTextColor(WHITE);    display.setCursor(3, 56);    display.println("Choose");    display.fillRect(48, 55, 41, 9, WHITE);    display.setTextSize(1);    display.setTextColor(BLACK);    display.setCursor(51, 56);    display.println("Delete");    display.setTextSize(1);    display.setTextColor(WHITE);    display.setCursor(101, 56);    display.println("Send");     display.display();    }    break;    case 2 :    {    display.fillRect(0, 55, 41, 9, BLACK); //choose    display.fillRect(48, 55, 45, 9, BLACK); //delete       display.setTextSize(1);    display.setTextColor(WHITE);    display.setCursor(3, 56);    display.println("Choose");    display.setTextSize(1);    display.setTextColor(WHITE);    display.setCursor(51, 56);    display.println("Delete");    display.fillRect(98, 55, 30, 9, WHITE);    display.setTextSize(1);    display.setTextColor(BLACK);    display.setCursor(101, 56);    display.println("Send");     display.display();    }    break;  } } int map(int potValue) {  if (potValue == 4095) {    return 36;  }  else  {  return int((potValue*37)/4095);  } }
When you are uploading to board A, you have to enter the MAC address of board B into that code at line 19. Also when you are uploading to board B, enter the MAC address of board A into that code at line 19.
After uploading the codes, you can use the,
- Potentiometer to select the character.
- Left button to….
- Right button to …